Now You Can Have Robust Data Logging for Free – Part 9
Computer Program Visual Basic (VB6) Continue
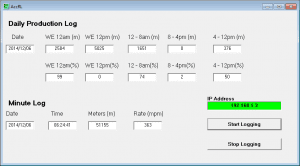
The final code will be completed for the program. If you want a link to download the complete code, go to the contact page and put a Robust Logger Program in the description.
The actual code that will be seen will be in italics. This way, you can pick out the code from the commentary.
MbusStatus = 0
ProductionPointerNow = 10
MinutePointerNow = 660
End Sub
Public MbusResponse As String
Dim MbusByteArray(255) As Byte
Public MbusStatus As Integer
Public ProductionPointer As Integer
Public ProductionPointerNow As Integer
Public MinutePointer As Integer
Public MinutePointerNow As Integer
Timer1.Enabled = False’ Stop the interval between reads to the PLC
Dim StartTime
If Winsock1.State <> 7 Then
If (Winsock1.State <> sckClosed) Then
Winsock1.Close
End If
Winsock1.RemoteHost = Text1.Text’ Set IP Address
Winsock1.Connect
StartTime = Timer’ Use the timer to determine if a connection cannot be made.
Do While ((Timer < StartTime + 2) And (Winsock1.State <> 7))
DoEvents
Loop
If (Winsock1.State = 7) Then
Text1.BackColor = &HFF00& ‘Change the background color to green
Else
Text1.BackColor = &HFF ‘Change the background color to red
Exit Sub
End If
End If
Select Case MbusStatus
Case 0, 2
‘Read all the Daily Production Values
MbusQuery = Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(6) + Chr(0) + Chr(3) + Chr((Val(ProductionPointerNow) \ 256)) + Chr(((Val(ProductionPointerNow) Mod 256) – 1)) + Chr(0) + Chr(20)
Case 3
‘Reset Daily Production Pointer to 30 (Write)
MbusQuery = Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(9) + Chr(1) + Chr(16) + Chr(0) + Chr(0) + Chr(0) + Chr(1) + Chr(2) + Chr(0) + Chr(30)
Winsock1.SendData MbusQuery
Case 4, 6
‘Read the Minute Log Pointer
MbusQuery = Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(6) + Chr(0) + Chr(3) + Chr(0) + Chr(1) + Chr(0) + Chr(1)
Case 5
‘Read all the Minute Log Values
MbusQuery = Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(6) + Chr(0) + Chr(3) + Chr((Val(MinutePointerNow) \ 256)) + Chr(((Val(MinutePointerNow) Mod 256) – 1)) + Chr(0) + Chr(20)
Case 7
‘Reset Minute Log Pointer to 670 (Write)
MbusQuery = Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(9) + Chr(1) + Chr(16) + Chr(0) + Chr(1) + Chr(0) + Chr(1) + Chr(2) + Chr(2) + Chr(158)
Winsock1.SendData MbusQuery
Case 8
‘Read the current Daily Production Values
MbusQuery = Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(6) + Chr(0) + Chr(3) + Chr(0) + Chr(9) + Chr(0) + Chr(20)
Case 9
‘Read the current Minute Log Values
MbusQuery = Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(0) + Chr(6) + Chr(0) + Chr(3) + Chr(2) + Chr(147) + Chr(0) + Chr(10) End Select
Winsock1.SendData MbusQuery ‘Send out the Modbus Information
Timer2.Enabled = True ‘Set the timeout timer for communications
Else
MsgBox (“Device not connected via TCP/IP”)
Exit Sub
End If
End Sub
Timer2.Enabled = False ‘Stop communications timeout timer
‘Get all the information from the data arriving
Dim B As Byte
Dim j As Byte
returnInfo = “”
For i = 1 To data length
Winsock1.GetData B
MbusByteArray(i) = B
returnInfo = returnInfo & B
Next
j = 0
On Error Resume Next
Select Case MbusStatus
Case 0
‘Read the Daily Production Pointer
ProductionPointer = Val(Str((MbusByteArray(10) * 256) + MbusByteArray(11)))
If ProductionPointer <> 30 Then
ProductionPointerNow = ProductionPointerNow + 20
MbusStatus = 1
Else
MbusStatus = 4
End If
Case 1
With Adodc1
.CommandType = adCmdTable
.RecordSource = “Production_Log”
.Refresh
.Recordset.AddNew
End With
‘Read all the Daily Production Values
Label18.Caption = Format$((Str((MbusByteArray(10) * 256) + MbusByteArray(11)) & “/” & Str((MbusByteArray(12) * 256) + MbusByteArray(13)) & “/” & Str((MbusByteArray(14) * 256) + MbusByteArray(15))), “yyyy/mm/dd”)
Label19.Caption = Val(Str((MbusByteArray(16) * 256) + MbusByteArray(17)) & Str((MbusByteArray(18) * 256) + MbusByteArray(19)))
Label20.Caption = Val(Str((MbusByteArray(20) * 256) + MbusByteArray(21)) & Str((MbusByteArray(22) * 256) + MbusByteArray(23)))
Label21.Caption = Val(Str((MbusByteArray(24) * 256) + MbusByteArray(25)) & Str((MbusByteArray(26) * 256) + MbusByteArray(27)))
Label22.Caption = Val(Str((MbusByteArray(28) * 256) + MbusByteArray(29)) & Str((MbusByteArray(30) * 256) + MbusByteArray(31)))
Label23.Caption = Val(Str((MbusByteArray(32) * 256) + MbusByteArray(33)) & Str((MbusByteArray(34) * 256) + MbusByteArray(35)))
Label24.Caption = Val(Str((MbusByteArray(36) * 256) + MbusByteArray(37))) / 10
Label25.Caption = Val(Str((MbusByteArray(38) * 256) + MbusByteArray(39))) / 10
Label26.Caption = Val(Str((MbusByteArray(40) * 256) + MbusByteArray(41))) / 10
Label27.Caption = Val(Str((MbusByteArray(42) * 256) + MbusByteArray(43))) / 10
Label28.Caption = Val(Str((MbusByteArray(44) * 256) + MbusByteArray(45))) / 10
With Adodc1
.Recordset.Update
.Recordset.MoveLast
.Refresh
End With ProductionPointerNow = ProductionPointerNow + 20
If ProductionPointer = ProductionPointerNow, Then
MbusStatus = 2
End If
Case 2
‘Read the Daily Production Pointer
ProductionPointer = Val(Str((MbusByteArray(10) * 256) + MbusByteArray(11)))
If ProductionPointer = ProductionPointerNow, Then
MbusStatus = 3
Else
ProductionPointerNow = ProductionPointerNow + 20
MbusStatus = 1
End If
Case 3
‘Reset Daily Production Pointer to 30 (Write)
If (MbusByteArray(8) = 16) And (MbusByteArray(12) = 1) Then
MbusStatus = 4
ProductionPointerNow = 10
Else
Text1.BackColor = &HFF
End If
With Adodc1
.CommandType = adCmdTable
.RecordSource = “Production_Log”
.Refresh
.Recordset.AddNew
End With
Case 4
‘Read the Minute Log Pointer
MinutePointer = Val(Str((MbusByteArray(10) * 256) + MbusByteArray(11)))
If MinutePointer <> 670 Then
MinutePointerNow = MinutePointerNow + 10
MbusStatus = 5
Else
MbusStatus = 8
End If
Case 5
With Adodc2
.CommandType = adCmdTable
.RecordSource = “Minute_Log”
.Refresh
.Recordset.AddNew
End With
‘Read all the Minute Values
Label29.Caption = Format$((Str((MbusByteArray(10) * 256) + MbusByteArray(11)) & “/” & Str((MbusByteArray(12) * 256) + MbusByteArray(13)) & “/” & Str((MbusByteArray(14) * 256) + MbusByteArray(15))), “yyyy/mm/dd”)
Label30.Caption = Format$((Str((MbusByteArray(16) * 256) + MbusByteArray(17)) & “:” & Str((MbusByteArray(18) * 256) + MbusByteArray(19)) & “:” & Str((MbusByteArray(20) * 256) + MbusByteArray(21))), “hh:nn:ss”)
Label31.Caption = Val(Str((MbusByteArray(22) * 256) + MbusByteArray(23)) & Str((MbusByteArray(24) * 256) + MbusByteArray(25)))
Label32.Caption = Val(Str((MbusByteArray(26) * 256) + MbusByteArray(27)))
With Adodc2
.Recordset.Update
.Recordset.MoveLast
.Refresh
End With MinutePointerNow = MinutePointerNow + 10
If MinutePointer <= MinutePointerNow, Then
MbusStatus = 6
End If
Case 6
‘Read the Minute Log Pointer
MinutePointer = Val(Str((MbusByteArray(10) * 256) + MbusByteArray(11)))
If MinutePointer = MinutePointerNow, Then
MbusStatus = 7
Else
MinutePointerNow = MinutePointerNow + 10
MbusStatus = 5
End If
Case 7
‘Reset Minute Log Pointer to 670 (Write)
If (MbusByteArray(8) = 16) And (MbusByteArray(12) = 1) Then
MbusStatus = 8
MinutePointerNow = 660
Else
Text1.BackColor = &HFF
End If
With Adodc2
.CommandType = adCmdTable
.RecordSource = “Minute_Log”
.Refresh
.Recordset.AddNew
.Refresh
End With
Case 8
‘Read the current Daily Production Values
Label18.Caption = Format$((Str((MbusByteArray(10) * 256) + MbusByteArray(11)) & “/” & Str((MbusByteArray(12) * 256) + MbusByteArray(13)) & “/” & Str((MbusByteArray(14) * 256) + MbusByteArray(15))), “yyyy/mm/dd”)
Label19.Caption = Val(Str((MbusByteArray(16) * 256) + MbusByteArray(17)) & Str((MbusByteArray(18) * 256) + MbusByteArray(19)))
Label20.Caption = Val(Str((MbusByteArray(20) * 256) + MbusByteArray(21)) & Str((MbusByteArray(22) * 256) + MbusByteArray(23)))
Label21.Caption = Val(Str((MbusByteArray(24) * 256) + MbusByteArray(25)) & Str((MbusByteArray(26) * 256) + MbusByteArray(27)))
Label22.Caption = Val(Str((MbusByteArray(28) * 256) + MbusByteArray(29)) & Str((MbusByteArray(30) * 256) + MbusByteArray(31)))
Label23.Caption = Val(Str((MbusByteArray(32) * 256) + MbusByteArray(33)) & Str((MbusByteArray(34) * 256) + MbusByteArray(35)))
Label24.Caption = Val(Str((MbusByteArray(36) * 256) + MbusByteArray(37))) / 10
Label25.Caption = Val(Str((MbusByteArray(38) * 256) + MbusByteArray(39))) / 10
Label26.Caption = Val(Str((MbusByteArray(40) * 256) + MbusByteArray(41))) / 10
Label27.Caption = Val(Str((MbusByteArray(42) * 256) + MbusByteArray(43))) / 10
Label28.Caption = Val(Str((MbusByteArray(44) * 256) + MbusByteArray(45))) / 10
With Adodc1
.Recordset.Update
.Recordset.MoveLast
End With MbusStatus = 9
Case 9
‘Read the current Minute Log Values
Label29.Caption = Format$((Str((MbusByteArray(10) * 256) + MbusByteArray(11)) & “/” & Str((MbusByteArray(12) * 256) + MbusByteArray(13)) & “/” & Str((MbusByteArray(14) * 256) + MbusByteArray(15))), “yyyy/mm/dd”)
Label30.Caption = Format$((Str((MbusByteArray(16) * 256) + MbusByteArray(17)) & “:” & Str((MbusByteArray(18) * 256) + MbusByteArray(19)) & “:” & Str((MbusByteArray(20) * 256) + MbusByteArray(21))), “hh:nn:ss”)
Label31.Caption = Val(Str((MbusByteArray(22) * 256) + MbusByteArray(23)) & Str((MbusByteArray(24) * 256) + MbusByteArray(25)))
Label32.Caption = Val(Str((MbusByteArray(26) * 256) + MbusByteArray(27)))
With Adodc2
.Recordset.Update
.Recordset.MoveLast
End With
MbusStatus = 0
End Select
Timer1.Enabled = True ‘Set the interval between the next communication
End Sub
Regards,
Garry
Now You Can Have Robust Data Logging for Free – Part 1
Now You Can Have Robust Data Logging for Free – Part 2
Now You Can Have Robust Data Logging for Free – Part 3
Now You Can Have Robust Data Logging for Free – Part 4
Now You Can Have Robust Data Logging for Free – Part 5
Now You Can Have Robust Data Logging for Free – Part 6
Now You Can Have Robust Data Logging for Free – Part 7
Now You Can Have Robust Data Logging for Free – Part 8
Now You Can Have Robust Data Logging for Free – Part 9
If you’re like most of my readers, you’re committed to learning about technology. Numbering systems used in PLCs are not challenging to learn and understand. We will walk through the numbering systems used in PLCs. This includes Bits, Decimals, Hexadecimal, ASCII, and Floating Points.
To get this free article, subscribe to my free email newsletter.
Use the information to inform other people how numbering systems work. Sign up now.
The ‘Robust Data Logging for Free’ eBook is also available as a free download. The link is included when you subscribe to ACC Automation.